Tagged template literals in ES2018
The first thing that was added to ES2018 was an improvement to a great feature called tagged template literals and it extends the templating that we already talked about in ES6. I never got around to working with this feature too much so this improvement to tagged template literals is a great opportunity to talk about the original feature.
So here’s a quick reminder about templating—using the backtick character `, I can do some templating and create text with variables. For example:
const name = "Moshe";
const age = 40;
const text = `${name} is ${age} years old`;
console.log(text); // "Moshe is 40 years old"
What the issue here? Imagine I want to add a qualifier to the age. For instance:
Moshe is 40 years old and young
Or, if he’s is 40 or older:
Moshe is 39 years old and he is dead in hightech.
How do I do this? Well, I could do something like this:
const name = 'Moshe';
const age = 40;
let remark = 'and young!'
if ( age >= 40) {
remark = 'and he is dead in hightech!'
}
const text = `${name} is ${age} years old ${remark}`;
console.log(text); // "Moshe is 40 years old and he is dead in hightech!"
But we’re aiming for encapsulation—in other words, that all parts of the code will do the same thing. If I also use templating like this, and the operation that adds text is part of the template, then why not put the whole thing inside a templating function? Then everything is in one place! That’s exactly what tagged templating does!
function myTemplate(strings, var1, var2) {
let remark = ' and young!'
if ( var2 >= 40) {
remark = ' and he is dead in hightech!'
}
return strings[0] + var1 + strings[1] + var2 + strings[2] + remark;
}
const name = 'Moshe';
const age = 40;
const text = myTemplate`Mr ${name} is ${age} years old`;
console.log(text); // // "Mr Moshe is 40 years old and young!"
And here’s a live example that you can mess around with:
Here too, in the templating function, I can pretty much do whatever I want. I can even not use variables at all! You can take it pretty far. Now, it’s not some insane function that we’ve never seen before—but it does help us gather everything connected to templating in one place and to not dirty up our functional code. Separating different parts of the code is important and makes life easier.
So what’s the big improvement in ES2018? Have a look at the following code:
function mySecondTemplate(strings, var1) {
let remark = '';
if (var1 > 3) {
remark = ' This is a lot!'
}
return strings[0] + var1 + strings[1] + remark;
}
const number = 4;
const text = mySecondTemplate`In my \c folder I have ${number} directories.`;
console.log(text); // "In my c folder I have 4 directories. This is a lot!"
document.write(text);
Yes, yes, it’s gonna’ work. Now let’s change it to \u:
function mySecondTemplate(strings, var1) {
let remark = '';
if (var1 > 3) {
remark = ' This is a lot!'
}
return strings[0] + var1 + strings[1] + remark;
}
const number = 4;
const text = mySecondTemplate`In my \u folder I have ${number} directories.`;
console.log(text); // NaN directories. This is a lot!
document.write(text);
Now we see the code is broken. Why? Because [u is the beginning of escaping in unicode and it’s not good script. The ES2018 proposal allows me to access the original value solely in the templating function:
function tag(strs) {
strs[0] === undefined
strs.raw[0] === "\\unicode";
}
tag`\unicode`
Using raw I can access the original value. And if this seems unnecessary to you, it’s a sign you’ve never written code in Node.js where you had to use slashes to carry out various actions.
But you know what? If you understood the tagged template literals, that’s probably good enough.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
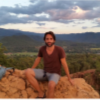
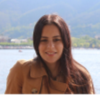
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment