Functions in TypeScript Part 1 — Parameters
In our last TypeScript article, we looked at loops. In this article, we’ll start looking at functions in TypeScript and dive deeper into parameters in TypeScript.
Functions are the essential ingredient in writing code that is readable, maintainable, and reusable. So what is a function? Functions are a group of statements whose purpose is to carry out a particular task. Functions are what we use to organize our program into logical blocks of code. Once a function has been defined, it can be called and the code can be accessed, making the code reusable.
Function declarations pass the function's name, return type, and parameters to the compiler. But the function definition is what constitutes the actual body of the function.
A function’s definition is what specifies what and how a particular task will be carried out. Before a function can be used, it must be defined. We do this with the function keyword—no surprise! Here is what a basic function definition looks like:
function () {
//function definition
console.log("function called")
}
But a function is no good until it’s been called. The syntax for that looks like this: Function_name(). Here’s a quick example:
function test() { // the definition
console.log("Let's call the function!")
}
test() // the invocation
Here’s is the JavaScript:
"use strict";
function test() {
console.log("Let's call the function!");
}
test(); // the invocation
And the output:
Let's call the function!
Something else functions can do is return values, along with control, back to the caller. These types of functions are known as returning functions. The syntax looks like this:
function function_name():return_type {
//statements
return value;
}
- The return_type may be any data type that is valid
- Returning functions must end in a return statement
- A function can return no more than one value i.e. there may be only one return statement per function
- The data type of the value returned needs to match the function’s return type
Have a look at this example:
//function defined
function greeting():string { //function will return a string
return "Hello World!"
}
function caller() {
var msg = greeting() //function greeting() invoked
console.log(msg)
}
//invoke function
caller()
- Above we declare the function greeting() with a return type of a string
- Line function returns a string value to the caller via the return statement
- The function greeting() returns a string, which gets stored in the variable msg. Later, this will be the output.
Here is the compiled JavaScript:
"use strict";
//function defined
function greeting() {
return "Hello World!";
}
function caller() {
var msg = greeting(); //function greeting() invoked
console.log(msg);
}
//invoke function
caller();
And the output:
Hello World!
TypeScript Parameters
Parameters are what we use to pass values to functions and form a part of the function’s signature. The parameter values get passed to the function when it is invoked. The number of values passed to a function needs to always match the number of parameters defined, unless it’s been explicitly specified.
When calling a function, there are two ways to pass arguments: calling by value or calling by pointer. The former method copies the value of the argument into a parameter of the function. In this case, changes made to the parameter inside the function will not have any effect on the argument. The latter is a method that copies the address of the argument into the formal parameter. Inside the function, that address can be used to access the actual argument that’s used in the call. Now let’s look at a few ways in which parameters are used by functions:
Positional Parameters
function func_name( param1 [:datatype], ( param2 [:datatype]) {
}
And here's our example:
function test_param(n1:number,s1:string) {
console.log(n1)
console.log(s1)
}
test_param(123,"this is a string")
- This block of code declares the function test_param with three parameters, n1, s1 and p1.
- It is not necessary to specify the parameter’s data type. If no data type is set, the parameter will be of the type any.
- The passed value’s data type must match that of the parameter during its declaration. If the data types don’t match, the compiler will throw an error.
Here is the JavaScript created, followed by the output to the compiler:
"use strict";
function test_param(n1, s1) {
console.log(n1);
console.log(s1);
}
test_param(456, "a string of text");
456
a string of text
Optional Parameters in TypeScript
When arguments don’t necessarily need to be passed for a function’s execution, this is a good time to use optional parameters. A parameter can be set as optional by adding a question mark to the name. An optional parameter should be the final argument in a function. The syntax to declare a function with an optional parameter is: function function_name (param1[:type], param2[:type], param3[:type])
Here’s an example:
function disp_details(id:number,name:string,mail_id?:string) {
console.log("ID:", id);
console.log("Name",name);
if(mail_id!=undefined)
console.log("Email",mail_id);
}
disp_details(789,"Arjun");
disp_details(555,"Lisa","lisa@abc.com");
- This example declares a parameterized function. Notice the third parameter mail_id. This is the optional parameter
- If no value is passed to an optional parameter during the function call, the parameter’s value will be undefined
- The function prints mail_id’s value of only if the argument is passed a value
Here is the JavaScript code:
"use strict";
function disp_details(id, name, mail_id) {
console.log("ID:", id);
console.log("Name", name);
if (mail_id != undefined)
console.log("Email", mail_id);
}
disp_details(789, "Arjun");
disp_details(555, "Lisa", "lisa@abc.com");
Finally, the output to the console:
ID: 789
Name Arjun
ID: 555
Name Lisa
Email lisa@abc.com
Rest Parameters in TypeScript
Rest parameters are a lot like variable arguments in Java. Rest parameters in TypeScript don’t limit the number of values that can be passed to a function. But, the the values that get passed must be of the same type. That is to say, rest parameters are like placeholders for multiple arguments of the same type.
To declare a rest parameter, put three periods before the name. Any parameters that are not rest parameters must precede the rest parameter.
Here is our example rest parameter:
function addNumbers(...nums:number[]) {
var i;
var sum:number = 0;
for(i = 0;i<nums.length;i++) {
sum = sum + nums[i];
}
console.log("The sum of the numbers is: ",sum)
}
addNumbers(2,3,4)
addNumbers(50,50,50,50,50)
- The function addNumbers() declaration, accepts a rest parameter nums. The rest parameter’s data type must be set to an array. Also, a function can have a maximum of one rest parameter
- The function is invoked twice, first by passing three values, then six
- The for loop iterates through the argument list passed to the function and calculates their sum
Here is the compiled JavaScript
"use strict";
function addNumbers(...nums) {
var i;
var sum = 0;
for (i = 0; i < nums.length; i++) {
sum = sum + nums[i];
}
console.log("The sum of the numbers is: ", sum);
}
addNumbers(2, 3, 4);
addNumbers(50, 50, 50, 50, 50);
And here is the result in the console:
The sum of the numbers is: 9
The sum of the numbers is: 250
Default Parameters
Function parameters in TypeScript may also be assigned default values. However, such parameters can also be explicitly passed values. Here's the syntax:
function function_name(param1[:type],param2[:type] = default_value) {
}
It’s important to note that parameters cannot be declared both optional and default at the same time. Here’s an example:
function calculate_discount(price:number,rate:number = 0.50) {
var discount = price * rate;
console.log("Discount Amount: ",discount);
}
calculate_discount(1000)
calculate_discount(1000,0.25)
Here is the JavaScript:
"use strict";
function calculate_discount(price, rate = 0.50) {
var discount = price * rate;
console.log("Discount Amount: ", discount);
}
calculate_discount(1000);
calculate_discount(1000, 0.25);
And the output to the console:
Discount Amount: 500
Discount Amount: 250
- This example declares a function called calculate_discount with two parameters: price and rate
- The value of the parameter rate is set to 0.50 by default.
- The program invokes the function, and passes only the value of the parameter price. Here, the value of rate is 0.50 (default)
- The same function is invoked, but with two arguments. The default value of rate is overwritten and is set to the value explicitly passed.
That’s all for this time. Check back soon and we’ll continue with more about functions in TypeScript, with anonymous functions, recursion, and more.
Recent Stories
Top DiscoverSDK Experts
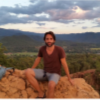
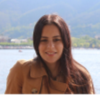
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment