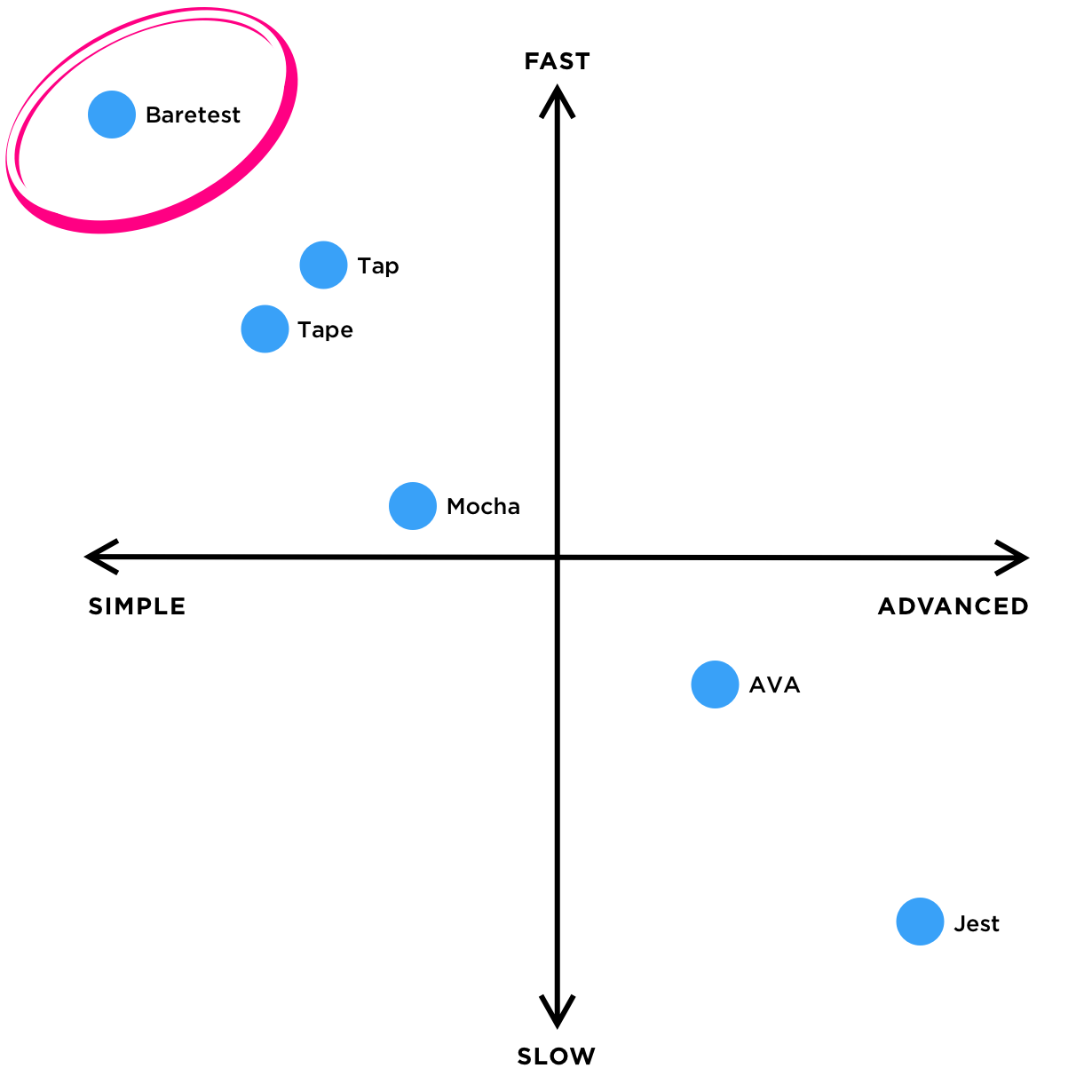
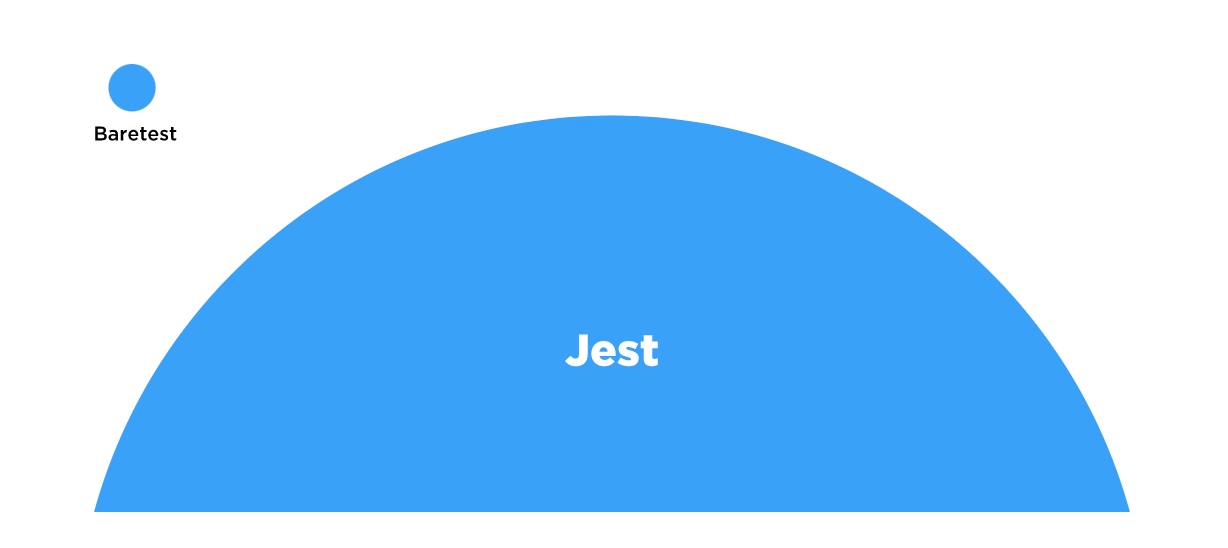
The package itself has just one main file and a couple of lines, which includes some functions; test, run, before, after, skip, and only. So, if you don’t like complexity, this might be for you.
TypeScript Support
Unfortunately, the package is only designed for JavaScript and doesn’t have typings. But with some minor adjustments (E.g. adding typings), we can also this in our TypeScript projects.
Github repo for Baretest typescript can be found here.
Setup and Getting Started
There’s a getting started documentation, but for our minimalistic TypeScript project our setup is as follows:
package.json
(dependencies)tsconfig.json
(config)sum.ts
(example code)test.ts
(tests)baretest.d.ts
(custom typings)
To get a good starting point for our TypeScript project we have a package.json
:
{
"name": "baretest-ts",
"version": "0.0.1",
"description": "Baretest in a TypeScript project example",
"repository": "https://github.com/jeroenouw/baretest-ts",
"license": "MIT",
"main": "./lib/test.js",
"keywords": [],
"scripts": {
"start": "npm run build && npm run quickstart",
"quickstart": "node ./lib/test.js",
"build": "npm run clean:min && tsc -p .",
"clean:min": "rm -rf ./lib",
"clean:max": "rm -rf ./node_modules ./package-lock.json ./lib",
"refresh": "npm run clean:max && npm install",
"tscov": "tscov --min-coverage 90",
"tscov:d": "tscov --details"
},
"dependencies": {
"tslib": "^1.11.1"
},
"devDependencies": {
"@liftr/tscov": "^1.4.4",
"@types/node": "^13.7.7",
"baretest": "^1.0.0",
"ts-node": "^8.8.1",
"typescript": "^3.8.3"
}
}
Notice we included the baretest
package as devDependency
. Also, we use the latest TypeScript version and have a package to see how many types we have covered: @liftr/tscov
. At last, we included some useful npm scripts.
Please run the next command:
npm install
Next, we need some basic config to run everything smoothly, tsconfig.json
:
{
"compilerOptions": {
"target": "ESNext",
"module": "commonjs",
"lib": ["ES2015", "ESNEXT", "dom"],
"outDir": "lib",
"rootDir": "src",
"types": [
"node",
],
"strict": true,
"esModuleInterop": true,
"importHelpers": true,
"noImplicitAny": false
},
"exclude": [
"node_modules",
]
}
Now we need some basic code which we can cover with some tests, ./src/sum.ts
:
const sum = (a: number, b: number): number => {
return a + b;
}export { sum };
Typings
To make the most out of TypeScript we, of course, need some typings. Because there are none yet available yet, we make them ourselves.
./src/typings/baretest.d.ts
:
export declare interface Baretest {
(t: string, f: Function): void;
run(): () => Promise<boolean>;
skip(): (f: Function) => void;
before(): (f: Function) => void;
after(): (f: Function) => void;
only(): (name: string, f: Function) => void;
}
Tests
Finally, we have a file called ./src/test.ts
. Here we import our dependencies and the basic sum
code. Next, we import the typings and use them in our new const called test
. There, we assign baretest which has one parameter where you can pass the title of our tests as an argument.
We added two basic tests that are correct and should succeed.
import baretest from 'baretest';
import assert from 'assert';
import { sum } from './sum';import { Baretest } from './typings/baretest';const test: Baretest = baretest('Baretest title');test('1 + 3', () => {
assert.equal(sum(1, 3), 4)
})test('2 + 3', () => {
assert.equal(sum(2, 3), 5)
})test.run()
To compile our TypeScript code and run baretest, we need to run:
npm start
We should quickly see this:
Baretest title • • ✓ 2
After compiling, we can also run the next command to immediately see our test results:
npm run quickstart
That’s it! The Github repo for Baretest typescript can be found here.